So the other day I was scrolling through my youtube feed & caught this episode of The Simple Programmer titled "Best Programming Languages of 2020".
I highly urge you to watch it. But if you're ok with spoilers, John's #1 recommendation is that instead of learning a new language, master one that you use. Smart huh?
Now I know it can be a bit difficult to not invest your free time in something new .. cause you know .. #fomo .. but I decided to spend a day getting into typescript with a bit of ecmascript.
Also, with COVID and isolation, instead of going through docs & manuals, I thought it might be fun to follow along a tutorial. Even if its long. Even if it starts with the basics. No harm in #backtobasics tho!
A few searches later, I landed on this tutorial..
I specifically liked this one cause instead of just a means to an end of developing an app, it had some good structure. Concepts were progressively introduced & one built on the other.
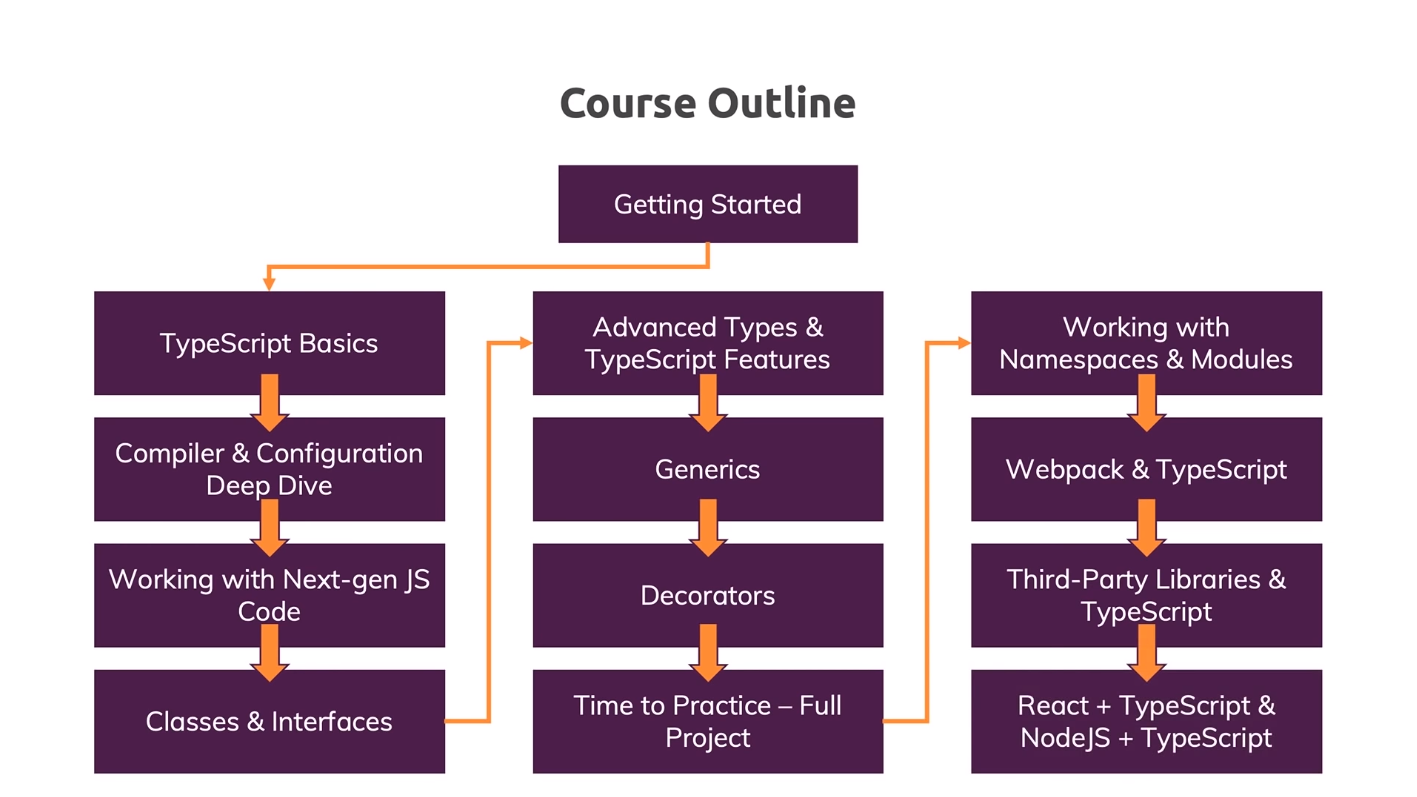
So I urge you to watch the video. What I'll be doing in this post is creating a cheat-sheet to synthesize it into something that is quickly referenceable. This is no way is an alternative to watching the video & trying all the examples tho! So don't be naughty. Put in the hard work. And as I love saying ... "Give it a go. Try it dev😉!" #ShamelessPlug.
Some important points for ES6
var
// (var) variables can be re-declared and updated
var x = 'hello';
var x = 'world'; // no error
if(true) {
var y = 'hey';
console.log(x); // returns 'world'
}
console.log(y); // returns 'hey' beacuse of hoisting.
// Note: Hoisting causes `var y;` to be added atop the file
// So `y` becomes globally available.
// It is declared BUT NOT INITIALISED!
let
// (let) can be updated but not re-declared
let x = 'hello';
let x = 'world'; // ERROR as can't redeclare
if(true) {
let y = 'hey';
}
console.log(y); // ERROR as y is scoped
const
// (const) cannot be updated or re-declared
const x = 'hello';
const x = 'world'; // ERROR as can't redeclare
if(true) {
const y = 'hey there';
x = '...'; // ERROR as can't update
}
console.log(y); // ERROR as y is scoped
It's all about those Types
basic types & functions
// Basic Types & Functions
const aNum: number = 1; // number type
const aStr: string = "hello world"; // string type
const aBool: boolean = true; // boolean type
const aDate: Date = new Date(); // object type
// Types & Functions
function doSomething(
aNumber: number,
aString: string,
aBoolean: boolean,
anOptionalParameter?: string
) {
// ...
}
// Call Function
doSomething(aNum, aStr, aBool); // OK
doSomething(aNum, aStr, aBool, aStr); // OK
//doSomething(aNum, aStr, aBool, aStr, aStr); // ERROR
fun with arrays
let stringArray: string[];
//stringArray = 'a'; // ERROR: Can't initialise as string
stringArray = ['a']; // OK
stringArray = ['a','b']; // OK
stringArray.push('c'); // OK
//stringArray.push(1); // ERROR: Can only push strings
console.log(stringArray); // Expect app.js:8 (3) ["a", "b", "c"]
let mixedArray: any[];
//mixedArray.push(1); // ERROR AT RUNTIME: array not initialised to push
mixedArray = []; // OK
mixedArray.push(1); // OK
mixedArray.push('a'); // OK
mixedArray.push(['a','b']); // OK
//mixedArray.push(null); // OK
mixedArray.push(true); // OK: But nothing is added to the array!
console.log(mixedArray); // Expect app.js:17 (4) [1, "a", Array(2), true]
let stringOrNumberArray: (string|number)[];
stringOrNumberArray = [];
stringOrNumberArray.push(1); // OK
stringOrNumberArray.push('a'); // OK
//stringOrNumberArray.push(true); // ERROR: must be number or string
console.log(stringOrNumberArray); // Expect app.js:23 (2) [1, "a"]
// Dealing with tuples (aka. fixed length array)
let kvp: [number, string];
kvp = [0, 'hey']; // OK
enums
// Dealing with Enums
enum Colors {
RED = 0,
BLUE,
GREEN,
}
let carColor: Colors = Colors.RED;
console.log(carColor); // Expect 0
console.log(Colors.BLUE); // Expect 1
console.log(Colors.GREEN); // Expect 2
enum Country {
AUSTRALIA = "AU",
UNITED_STATES = "US",
}
console.log(Country.AUSTRALIA); // Expect AU
console.log(Country.UNITED_STATES); // Expect US
any
, union
, literal
// Dealing with other types
// any type (meh, don't care)
let x: any;
x = 0; // OK
x = 'a'; // OK
x = true; // OK
// union type (can be of type a OR b)
let y: number | string;
y = 0; // OK
y = 'a'; // OK
//y = true; // ERROR - 'true' is not assignable to type 'string | number'
// literal type (must be exactly one of these ...)
let z: 'a'| 0;
z = 0; // OK
z = 'a'; // OK
//z = true; // ERROR - 'true' is not assignable to type 'string | number'
Functions
Declarations
Some different ways of declaring them ...
// Fun with functions
// Option 1
let doSomething = function (input: string) {
return `Do something with ${input}`;
}
console.log(doSomething('this.'));
// Option 2
doSomething = (input: string) => {
return `Do something with ${input}`;
}
console.log(doSomething('this..'));
// Option 3
doSomething = (input: string) => `Do something with ${input}`;
console.log(doSomething('this...'));
// Option 4
doSomething = (input: string) : string => `Do something with ${input}`;
console.log(doSomething('this....'));
// Option 5
doSomething = input => `Do something with ${input}`;
console.log(doSomething('this....'));
Other concepts
arrays
, spread operator
, object destructuring
// arrays of specific types can be added
type Movie = { title: string };
let movies: Movie[] = [{ title: `Zoolander` }];
console.log(movies); // app.js:9 [{…}]
// use the spreadoperator to clone items
let addMovie = (title: string) => (movies = [...movies, { title }]);
addMovie(`Emoji Movie`); // app.js:12 (2) [{…}, {…}]
console.log(movies);
type Car = {
make: string;
model: string;
};
let myCar: Car = { make: "VW", model: "Golf" };
var { make, model } = myCar;
console.log(make);
console.log(model);
type
v/s interface
// Custom Type Definition
// via type
type Person = {
name: string;
age: number;
introductions: () => string;
};
// via interfaces
interface IPerson {
name: string;
age: number;
introductions: () => string;
}
// benefit of using interface is that they're extensible
interface IEmployee extends IPerson {
employeeId: number;
}
// type is not extensible
// type Student extends Person = {}; // ERROR:
// types can refer to other types/interfaces
type emp = IEmployee;
// Using types with classes
class WorkShift {
teamLeader: emp;
constructor(teamLeader: emp) {
this.teamLeader = teamLeader;
}
}
var morningShift = new WorkShift({
name: "Jake",
age: 20,
employeeId: 1001,
introductions: () => `Hi there!`,
});
console.log(morningShift.teamLeader.name); // Expect Jake